JavaScript Web Scraping Guide: Methods & Tools (2024)
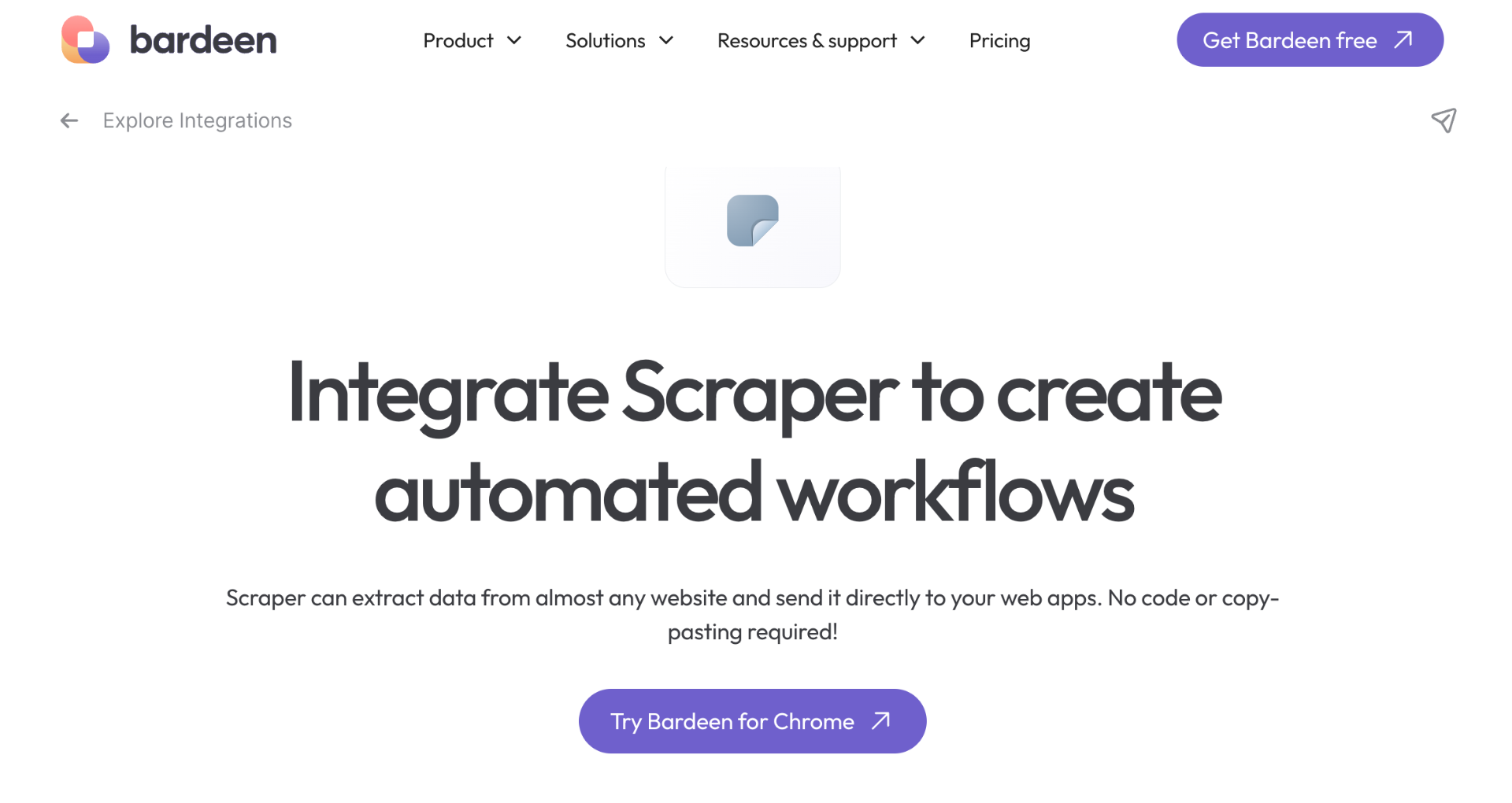
TL;DR
Web scraping with JavaScript is a process for extracting data from websites, utilizing tools like Node.js, Axios, Cheerio, and Puppeteer. It requires understanding of JavaScript, Node.js, and HTML DOM. The guide includes step-by-step tutorials for scraping static and dynamic content, emphasizing ethical practices.
Learn to scrape data efficiently and responsibly.
Enhance your data collection and analysis process by incorporating Bardeen's automation capabilities into your workflow.
Web scraping, the process of extracting data from websites, is a powerful technique that enables you to gather information efficiently. JavaScript, being a versatile programming language, provides various tools and libraries to make web scraping tasks easier, both on the client-side and server-side. In this step-by-step tutorial, we'll guide you through the process of web scraping using JavaScript, covering essential concepts, tools, and practical examples to help you master the art of extracting data from the web in 2024.
Introduction to Web Scraping with JavaScript
Web scraping is the process of automatically extracting data from websites. It's a powerful technique that enables you to gather information from the vast amount of data available on the internet. JavaScript, being a versatile programming language, provides various tools and libraries to make web scraping tasks easier and more efficient.
Here are some key points about web scraping with JavaScript:
- JavaScript can be used for both client-side and server-side web scraping
- Client-side scraping involves running JavaScript code in the browser to extract data from web pages
- Server-side scraping utilizes JavaScript libraries and frameworks like Node.js to scrape data from websites
- JavaScript provides powerful libraries such as Puppeteer and Cheerio that simplify the web scraping process
.png)
Whether you need to collect data for analysis, monitor prices, or automate tasks, web scraping with JavaScript offers a flexible and efficient solution. In the following sections, we'll explore the techniques and tools you can use to scrape websites using JavaScript effectively.
Setting Up Your JavaScript Environment
To begin web scraping with JavaScript, you'll need to set up a Node.js environment. Node.js allows you to run JavaScript code outside of a web browser, making it ideal for server-side scripting and web scraping tasks. Here's how to get started:
.png)
- Install Node.js: Visit the official Node.js website (https://nodejs.org) and download the appropriate version for your operating system. Follow the installation instructions provided.
- Verify the installation: Open a terminal or command prompt and run the following command to check if Node.js is installed correctly:
node -v
If Node.js is installed, it will display the version number. - Create a new project directory: Choose a location on your computer where you want to store your web scraping project. Create a new directory and navigate to it using the terminal or command prompt.
- Initialize a new Node.js project: Run the following command to initialize a new Node.js project in your project directory:
npm init -y
This command will create apackage.json
file with default settings. - Install required dependencies: For web scraping, you'll typically need libraries like Axios for making HTTP requests and Cheerio for parsing HTML. Install them using the following commands:
npm install axios cheerio
With these steps completed, you now have a Node.js environment set up and ready for web scraping. You can create a new JavaScript file (e.g., scraper.js
) and start writing your web scraping code using the installed dependencies.
Bardeen can save you time by automating repetitive tasks. Try using this scraping integration to focus on the important work.
.png)
Understanding HTTP Requests and Responses
Web scraping relies heavily on making HTTP requests to servers and handling the responses that are sent back. Let's dive into the fundamentals of HTTP requests and responses.
An HTTP request is made by a client, typically a web browser, to a server to request a specific resource or perform an action. The request includes the URL of the resource, the HTTP method (GET, POST, etc.), and optional headers and data.
The server processes the request and sends back an HTTP response. The response includes a status code indicating the result of the request (e.g., 200 for success, 404 for not found), headers containing metadata about the response, and the requested data in the response body.
When web scraping with JavaScript, you can use different methods to make HTTP requests:
- Fetch API: The Fetch API is a modern, promise-based way to make asynchronous HTTP requests. It provides a clean and concise syntax for sending requests and handling responses.
- Axios: Axios is a popular JavaScript library that simplifies making HTTP requests. It supports promises, request and response interceptors, and automatic transformation of request and response data.
Here's a simple example using the Fetch API to make a GET request:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => console.log(data)) .catch(error => console.error(error));
In this example, the fetch()
function is used to send a GET request to the specified URL. The response is then parsed as JSON using response.json()
, and the resulting data is logged to the console. Any errors that occur during the request are caught and logged as well.
Understanding how to make HTTP requests and handle responses is crucial for effective web scraping. By leveraging the Fetch API or libraries like Axios, you can easily retrieve data from web pages and APIs, enabling you to extract and process the information you need.
Utilizing Puppeteer for Dynamic Web Scraping
Puppeteer is a powerful Node.js library that allows you to automate and control a headless Chrome or Chromium browser. It provides an API to navigate web pages, interact with elements, and extract data from websites, making it an excellent tool for dynamic web scraping.
.png)
Here's a basic example of using Puppeteer to navigate to a page, render JavaScript, and scrape the resulting data:
const puppeteer = require('puppeteer');(async () => {const browser = await puppeteer.launch();const page = await browser.newPage();await page.goto('https://example.com');await page.waitForSelector('#content');const data = await page.evaluate(() => {return document.querySelector('#content').innerText;});console.log(data);await browser.close();})();
In this example:
- We launch a new browser instance using
puppeteer.launch()
. - We create a new page with
browser.newPage()
. - We navigate to the desired URL using
page.goto()
. - We wait for a specific selector to be available using
page.waitForSelector()
. - We use
page.evaluate()
to execute JavaScript code within the page context and extract the desired data. - Finally, we close the browser with
browser.close()
.
Puppeteer provides many other useful methods for interacting with web pages, such as:
page.click()
to simulate clicking on elements.page.type()
to simulate typing into form fields.page.screenshot()
to capture screenshots of the page.page.pdf()
to generate PDF files from the page.
By leveraging Puppeteer's capabilities, you can handle dynamic content, perform actions on the page, and extract data that may not be easily accessible through static HTML parsing.
Bardeen can save you time by automating repetitive tasks. Try using this scraping integration to focus on the important work.
Static Data Extraction with Cheerio
Cheerio is a powerful library that allows you to parse HTML documents on the server-side using a syntax similar to jQuery. It provides an easy way to extract specific elements and data from static web pages.
.png)
Here's a step-by-step example of scraping a static site using Cheerio:
- Install Cheerio using npm:
npm install cheerio
- Load the HTML document:
const cheerio = require('cheerio');const $ = cheerio.load(html);
- Use Cheerio selectors to target specific elements:
const title = $('h1').text();const paragraphs = $('p').map((i, el) => $(el).text()).get();
- Extract the desired data:
console.log(title);console.log(paragraphs);
In this example, we use Cheerio to load the HTML document and then use selectors to extract the text content of the \u003ch1\u003e
element and all \u003cp\u003e
elements. The map()
function is used to iterate over the selected \u003cp\u003e
elements and extract their text content.
Cheerio provides a wide range of selectors and methods to navigate and manipulate the parsed HTML document, making it easy to extract specific data from static web pages.
Handling Pagination and Multi-page Scraping
When scraping websites with pagination, you need to handle navigating through multiple pages to extract all the desired data. Here are some techniques to handle pagination in JavaScript:
- Identify the pagination pattern:
- Look for "Next" or "Page" links in the HTML structure.
- Analyze the URL pattern for paginated pages (e.g.,
/page/1
,/page/2
).
- Implement a loop or recursive function:
- Use a loop to iterate through the pages until a specific condition is met (e.g., no more "Next" link).
- Recursively call the scraping function with the URL of the next page until all pages are processed.
- Extract data from each page:
- For each page, make an HTTP request to fetch the HTML content.
- Use Cheerio or Puppeteer to parse and extract the desired data from the page.
- Store the extracted data in a suitable format (e.g., array, object).
Here's an example of a recursive function to scrape paginated data:
async function scrapePaginated(url, page = 1) {
const response = await fetch(`${url}?page=${page}`);
const html = await response.text();
const $ = cheerio.load(html);
// Extract data from the current page
const data = extractData($);
// Check if there is a next page
const nextPageLink = $('a.next-page').attr('href');
if (nextPageLink) {
// Recursively call the function with the next page URL
const nextPageData = await scrapePaginated(url, page + 1);
return [...data, ...nextPageData];
}
return data;
}
In this example, the scrapePaginated
function takes the base URL and the current page number as parameters. It fetches the HTML content of the current page, extracts the data using Cheerio, and checks if there is a next page link. If a next page exists, it recursively calls itself with the next page URL. Finally, it combines the data from all pages and returns the result.
By implementing pagination handling, you can ensure that your web scraper retrieves data from all relevant pages, enabling comprehensive data extraction from websites with multiple pages.
You can save time by using Bardeen to automate scraping tasks. Try this web scraper to simplify your workflow.
Data Storage and Management
After scraping data from websites, you need to store and manage it effectively for further analysis or usage. Here are some options for storing and managing scraped data in JavaScript:
- JSON files:
- Save the scraped data as a JSON file using the built-in
fs
module in Node.js. - JSON provides a structured and readable format for storing data.
- Example:
const fs = require('fs');
const scrapedData = [/* your scraped data */];
fs.writeFile('data.json', JSON.stringify(scrapedData), (err) => {
if (err) throw err;
console.log('Data saved to data.json');
});
- Save the scraped data as a JSON file using the built-in
- Databases:
- Store the scraped data in a database for efficient querying and management.
- Popular choices include MongoDB (NoSQL) and MySQL (SQL).
- Use a database driver or ORM (Object-Relational Mapping) library to interact with the database from Node.js.
- Example with MongoDB:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost/scraperdb', { useNewUrlParser: true });
const dataSchema = new mongoose.Schema({
// define your data schema
});
const DataModel = mongoose.model('Data', dataSchema);
const scrapedData = [/* your scraped data */];
DataModel.insertMany(scrapedData)
.then(() => console.log('Data saved to MongoDB'))
.catch((err) => console.error('Error saving data:', err));
- CSV files:
- If your scraped data is tabular, you can save it as a CSV (Comma-Separated Values) file.
- Use a CSV library like
csv-writer
to create and write data to CSV files. - Example:
const createCsvWriter = require('csv-writer').createObjectCsvWriter;
const csvWriter = createCsvWriter({
path: 'data.csv',
header: [
{ id: 'name', title: 'Name' },
{ id: 'age', title: 'Age' },
// ...
]
});
const scrapedData = [/* your scraped data */];
csvWriter.writeRecords(scrapedData)
.then(() => console.log('Data saved to data.csv'))
.catch((err) => console.error('Error saving data:', err));
When choosing a storage method, consider factors such as the size of your scraped data, the need for querying and analysis, and the ease of integration with your existing infrastructure.
Additionally, ensure that you handle data responsibly and securely, especially if you're dealing with sensitive or personal information. Implement appropriate access controls, encryption, and data protection measures to safeguard the scraped data.
By storing and managing scraped data effectively, you can leverage it for various purposes, such as data analysis, machine learning, or building applications that utilize the extracted information.
Legal and Ethical Considerations of Web Scraping
When engaging in web scraping, it's crucial to be aware of the legal and ethical considerations to ensure responsible data collection practices. Here are some key points to keep in mind:
- Respect website terms of service and robots.txt:
- Always review and comply with the website's terms of service and robots.txt file.
- robots.txt is a file that specifies which parts of the website are allowed or disallowed for scraping.
- If a website explicitly prohibits scraping in their terms of service or robots.txt, respect their guidelines and refrain from scraping.
- Avoid overloading servers:
- Scrape websites responsibly by controlling the rate of requests sent to the server.
- Sending too many requests in a short period can overload the server and disrupt the website's functionality.
- Implement delays between requests and avoid aggressive scraping that can be perceived as a denial-of-service attack.
- Be transparent and identify yourself:
- Use a clear user agent string that identifies your scraper and provides a way for website owners to contact you.
- This transparency helps website owners understand the purpose of your scraping and reach out if there are any concerns.
- Respect intellectual property rights:
- Be mindful of copyright and intellectual property rights when scraping and using the collected data.
- Ensure that your scraping and subsequent use of the data comply with applicable laws and regulations.
- Protect user privacy:
- If you collect personal or sensitive information during scraping, handle it responsibly and in compliance with data protection regulations like GDPR or CCPA.
- Anonymize or remove personally identifiable information if necessary.
- Use scraped data ethically:
- Ensure that the scraped data is used for legitimate and ethical purposes.
- Avoid using scraped data for spamming, unauthorized marketing, or any activities that violate privacy or cause harm.
To ensure ethical web scraping, consider the following best practices:
- Use APIs provided by websites whenever possible instead of scraping.
- Limit the scraping frequency and respect website resources.
- Comply with any request from website owners to stop scraping if they raise concerns.
- Consult with legal experts to ensure compliance with applicable laws and regulations.
By adhering to these legal and ethical guidelines, you can engage in web scraping responsibly, maintain a positive relationship with website owners, and avoid potential legal issues. Remember, the key is to strike a balance between collecting valuable data and respecting the rights and interests of website owners and users.
You can save time by using Bardeen to automate scraping tasks. Try this web scraper to simplify your workflow.
Automate Web Scraping with Bardeen Playbooks
Web scraping with JavaScript allows for the automated extraction of data from websites, which can significantly enhance your data collection processes for analytics, market research, or content aggregation. While manual scraping methods are effective for small-scale projects, automating the web scraping process can save time and increase efficiency, especially when dealing with large volumes of data.
Bardeen, with its powerful automation capabilities, simplifies the web scraping process. Utilizing Bardeen's playbooks, you can automate data extraction from various websites into platforms like Google Sheets, Notion, and more without writing a single line of code.
- Extract information from websites in Google Sheets using BardeenAI: This playbook automates the extraction of any information from websites directly into a Google Sheet, streamlining the process of gathering data for analytics or market research.
- Get keywords and a summary from any website save it to Google Sheets: Automate the extraction of data from websites, create brief summaries, identify keywords, and store the results in Google Sheets. Ideal for content creators and marketers looking to analyze web content efficiently.
- Scrape and Save Google Search Results into Notion: This workflow automates the process of searching Google, scraping the search results, and saving them into a Notion database, perfect for market research and competitor analysis.
By leveraging these Scraper playbooks, you can automate the tedious task of web scraping, allowing you to focus on analyzing the data. Enhance your data collection and analysis process by incorporating Bardeen into your workflow.
Learn how to find or recover an iCloud email using a phone number through Apple ID recovery, device checks, and email searches.
Learn how to find someone's email on TikTok through their bio, social media, Google, and email finder tools. A comprehensive guide for efficient outreach.
Learn how to find a YouTube channel's email for business or collaborations through direct checks, email finder tools, and alternative strategies.
Learn how to find emails on Instagram through direct profile checks or tools like Swordfish AI. Discover methods for efficient contact discovery.
Learn why you can't find Reddit users by email due to privacy policies and discover 3 indirect methods to connect with them.
Learn how to find someone's email address for free using reverse email lookup, email lookup tools, and social media searches. A comprehensive guide.
Your proactive teammate — doing the busywork to save you time
Integrate your apps and websites
Use data and events in one app to automate another. Bardeen supports an increasing library of powerful integrations.
Perform tasks & actions
Bardeen completes tasks in apps and websites you use for work, so you don't have to - filling forms, sending messages, or even crafting detailed reports.
Combine it all to create workflows
Workflows are a series of actions triggered by you or a change in a connected app. They automate repetitive tasks you normally perform manually - saving you time.
Don't just connect your apps, automate them.
200,000+ users and counting use Bardeen to eliminate repetitive tasks